Introduction
Developers frequently bump into different c# collection interfaces, which can sometimes be a head-scratcher. The big three in this mix are IEnumerable
, ICollection
, and IList
. Today, we’ll break down these interfaces, making them easy to understand. In addition to this, I’ll highlight the key differences between using IEnumerable
and List
. Let’s get started!
Interface hierarchy
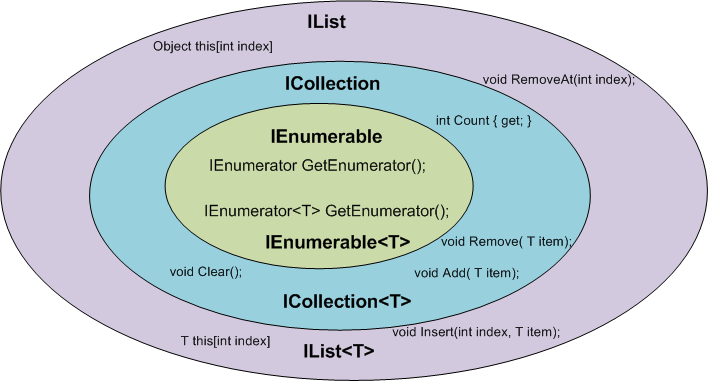
1. IEnumerable
Definition: IEnumerable
is the most basic interface that represents a forward-only cursor of T
. Any collection that can be iterated using a foreach
loop in C# implements this interface.
Example:
IEnumerable<string> names = new List<string>() { "Alice", "Bob", "Charlie" };
foreach (var name in names)
{
Console.WriteLine(name);
}
Key Points:
- It provides a basic iteration capability.
- It doesn’t support adding or removing items.
- It doesn’t provide random access to items.
2. ICollection
Definition: ICollection
is a step up from IEnumerable
. It includes all the capabilities of IEnumerable
and adds functionalities like adding, removing, and checking the existence of items.
Example:
ICollection<string> names = new List<string>() { "Alice", "Bob" };
names.Add("Charlie");
names.Remove("Alice");
Console.WriteLine(names.Contains("Bob")); // Outputs: True
Key Points:
- It extends
IEnumerable
. - Provides methods to add and remove items.
- Can check the number of items and if a particular item exists.
3. IList
Definition: IList
is an extension of ICollection
that provides capabilities to access elements by index, check the index of a particular item, and insert or remove items at a specific position.
Example:
IList<string> names = new List<string>() { "Alice", "Charlie" };
names.Insert(1, "Bob");
Console.WriteLine(names[1]); // Outputs: Bob
Key Points:
- It extends both
IEnumerable
andICollection
. - Supports indexed access.
- Can insert or remove items at a specific position.
IEnumerable vs. List
While IEnumerable
is an interface that provides basic iteration capabilities, List
is a concrete implementation that offers a set of functionalities, including adding, removing, and accessing items by index.
Key Differences:
IEnumerable
is read-only, whileList
allows modification.List
provides faster access to items by index.IEnumerable
is more suitable for scenarios where only iteration is required, whereasList
is versatile and can be used in a wider range of scenarios.
Example:
List<string> myList = new List<string>() { "Alice", "Bob", "Charlie" };
myList.Add("David");
IEnumerable<string> myEnumerable = myList;
Conclusion
Understanding the nuances between IEnumerable
, ICollection
, and IList
is very important for us C# developers. While they might seem similar, each serves a unique purpose. By grasping their differences and when to use each, we can write more efficient and cleaner code.
I hope this article provides a clear understanding of the differences between IEnumerable
, ICollection
, and IList
, as well as the distinction between IEnumerable
and List
.
If you have further questions or need more examples, feel free to ask!
Happy coding!