Clean Architecture in .NET has become a vital concept for modern software development. It promotes maintainability, testability, and scalability, making it a popular choice among C# developers. In this guide, we’ll explore the principles of Clean Architecture and provide practical C# examples to help you implement it in your .NET projects.
What is Clean Architecture in .NET?
Clean Architecture is a design philosophy that emphasizes separation of concerns and loosely-coupled components. It allows developers to create flexible and maintainable code by organizing the codebase into different layers. In the context of .NET, Clean Architecture is often implemented using C#, following specific principles and patterns.
Key Principles of Clean Architecture
- Independence of Frameworks: The architecture does not depend on specific libraries or frameworks.
- Testable: The business logic can be tested without the UI, database, or any external elements.
- Independence of UI: The UI can change without affecting the rest of the system.
- Independence of Database: The database and business logic are decoupled.
Diagram of Clean Architecture
- Innermost Circle (Domain): Represents the core business logic and domain entities.
- Second Circle (Application): Contains application-specific business rules and use cases.
- Third Upper Half Circle (Presentation): Houses the interfaces, UI, and controllers.
- Third Lower Half Circle (Infrastructure and Persistence): Includes external concerns like databases, and frameworks, and any third party tool integrated into the system
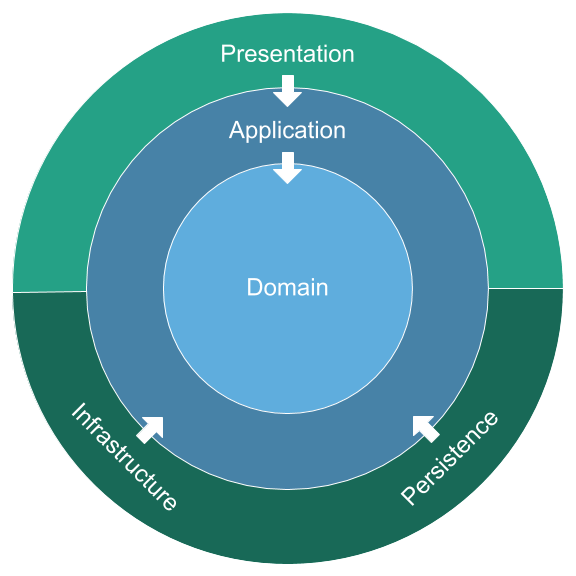
The dependencies point inward, meaning that inner circles are independent of the outer ones.
Implementing Clean Architecture in .NET with C# Examples
1. Creating the Domain Layer
The Domain Layer contains the core business logic and entities. Here’s a simple C# example of a domain entity:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
2. Designing the Application Layer
The Application Layer defines the use cases and interfaces. Here’s an example of a C# interface for a product repository:
public interface IProductRepository
{
Product GetById(int id);
}
public class ProductService: IProductRepository
{
private readonly IProductRepository _repository;
public ProductService(IProductRepository repository)
{
_repository = repository;
}
public Product GetById(int id)
{
return _repository.GetById(id);
}
}
3. Building the Infrastructure Layer
This layer contains the implementation details, such as database access. Here’s a C# example using Entity Framework:
public class ProductRepository : IProductRepository
{
private readonly DbContext _context;
//...//
public Product GetById(int id) => _context.Products.Find(id);
}
4. Developing the Presentation Layer
The Presentation Layer handles the UI and user interaction, this could be a Frontend application or a RESTful WebAPI Project. Here’s a C# example of a simple controller in ASP.NET Core:
public class ProductController : Controller
{
private readonly IProductRepository _repository;
public ProductController(IProductRepository repository)
{
_repository = repository;
}
public IActionResult Details(int id)
{
var product = _repository.GetById(id);
return View(product);
}
}
When to Use and When Not to Use Clean Architecture
When to Use
- Complex Applications: When building large-scale applications with multiple modules and features.
- Long-Term Projects: In projects that require maintainability and scalability over time.
- Collaborative Development: When multiple teams are working on different parts of the application.
When Not to Use
- Simple, Short-Term Projects: Overhead might outweigh the benefits for small or temporary projects.
- Tight Deadlines: Implementing Clean Architecture takes time and might not be suitable for projects with aggressive timelines.
- Inexperienced Team with the Architecture: If the team is unfamiliar with Clean Architecture, the learning curve might slow down development.
Conclusion: Embracing Clean Architecture in .NET
Clean Architecture in .NET offers a structured approach to software development. By understanding its principles and knowing when to apply them, you can create resilient and adaptable applications. The C# examples provided here serve as a starting point for implementing Clean Architecture in your .NET projects, allowing you to harness its benefits and navigate its challenges.
Happy coding!