Improve your .NET Skills
Unleash your potential as a Software Developer! In this site you will find a ton of practical exercises so you can increase your knowledge not only in logical concepts, but also design patterns, best coding practices, architectural patterns, and much more! And all of this absolutely for free!
SOFTWARE ARCHITECTURE
Apply latest trends in Software Architecture. Clean Architecture, Vertical Slices, and more
DESIGN PATTERNS
Familiarize yourself with Design Patterns, and understand in detail when and why to use them
BEST CODING PRACTICES
The name says it all, improve your coding with the best and most trending practices
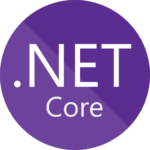
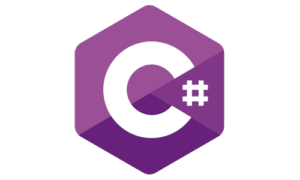

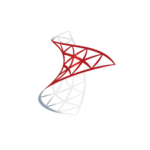
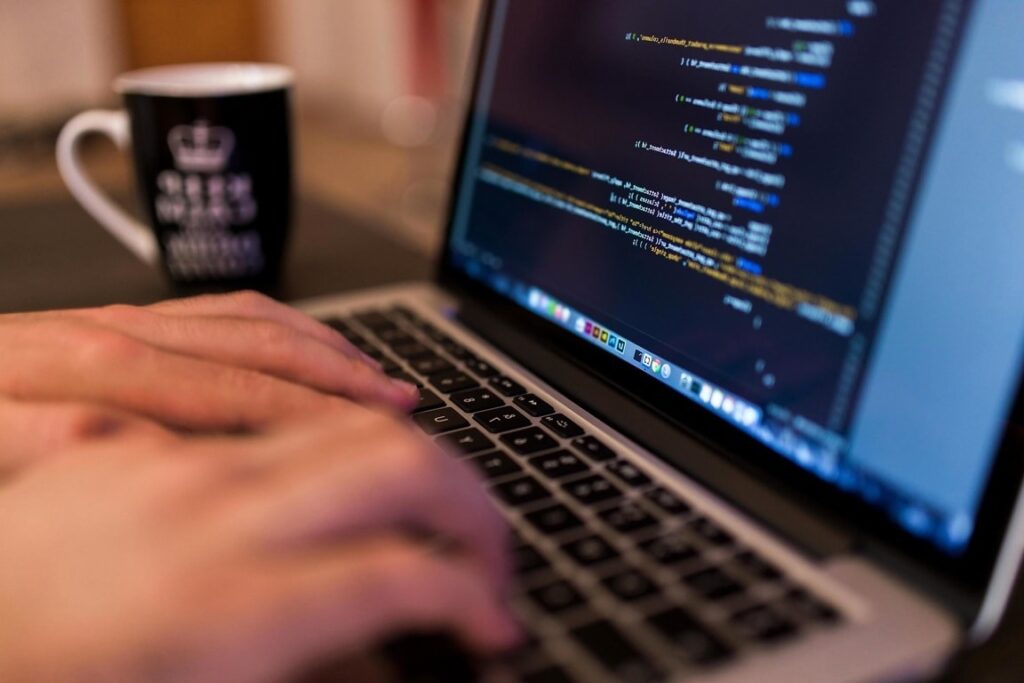
Join our developer community and get an actionable exercise sent to your inbox on a weekly basis!
Stay up to date with the latest content.
Your subscription could not be saved. Please try again.
Your subscription has been successful. Welcome aboard!
Latest Exercises
29 August 2023If you followed along the series of exercises I’ve been sharing in this website, our previous exercise was intended to create a Console Application (with interesting complexities) to be used for CRUD operations on a Book Library shop.
If you missed it, please make sure to have a look here: C# Exercise – Book Library Console Application
In today’s exercise, we will extend this existing solution, with a new layer which will act as a RESTful API. It will be a .NET WebAPI project with the proper endpoints and the ability to reuse all the architecture that is already set on the solution.
If you didn’t practice on the previous exercise don’t worry, just grab the example I’ve coded in my Github HERE , it’s ready to use.
Requirements and Challenges in this exercise
Extend an existing solution, without modifying anything from the architecture.
Implement a RESTful API approach using a Controller with the proper Endpoints and reuse the existing BookService to get access to the CRUD operations.
For the purpose of this exercise use sync methods on the controller
Enhance the solution with Logging capabilities (Custom made, Serilog, etc. Your choice)
Setting up a Docker image, so we deploy the App into a container, and run it there (Use default ports to browse the API inside the container)
Push the enhanced solution into your Github profile. (GIT Basics here)
Optional Challenges
If you want to go even further making use of this existing exercise, I propose the following:
Upgrade the Controller to run async methods. All implementation should be improved to handle async requests
Implement MediatR with CQRS to handle the requests. Popular and modern approach with API development these days.
Move the Console App as “Legacy” code, yet functional but clearly visible on the solution as an old and unused approach, close to decommission. BookService is also targeted as Legacy code, the new approach using handlers will replace the service with a modern approach.
Implement basic validation with FluentValidation
The Solution
As usual, you can find my own implementation in my Github profile, as a way to compare your code and check my approach for the exercise. You can also use it to validate your code and get inspiration, if you get stuck at some point. But as a rule of thumb, always try to implement your own solution first, and get used to investigate problems before seeking for an existing approach to review. This is what will make a better developer out of you, a problem solver.
Repo:Â https://github.com/marcelomusza/LibraryExerciseAPIWithDocker
In addition to this, if you went even further with the optional challenges, I have a private repository for the proposed solution, accessible only by my Patreons. If you are interested in contributing with an active community of developers, and helping with the support of this project, please consider subscribing! We’ll love to have you onboard.
For more information about Patreon, click here.
The private Repo: https://github.com/marcelomusza/LibraryExerciseAPIWithDocker_Private
See you on the next Exercise, happy coding! [...]
Read more...
16 August 2023Let’s practice today a series of concepts in this simple Console Application. But beware that it won’t be as simple as it looks 🙂
This exercise will put your hands on a book library solution, which needs to have a UI using a simple Console application. But in the guts of the application, the expectation is to have a layered architecture, a Service that will interact with these books, and a valid database connection (using an InMemory database this time) through Repositories. We will publish and deploy this Application into a Docker container, and lastly, we’ll push this code into a Git repository.
Challenges in this exercise:
Set up a Console Application that will act as the UI of the solution
Layered Architecture (Clean Architecture)
Use of the Repository Pattern for adding/updating/listing/removing books
Connection to an InMemory Database using Entity Framework.
Seed 5 books to this database
Correct usage of IEnumerable, ICollection, IList, and Lists wherever applicable
Setting up a Docker image, so we deploy the App into a container, and run it there
Push your code into a new Git repository
Requirements:
Entities Layer:
Create a Book class with properties: Id, Title, Author, and Rating.
public class Book
{
public int Id { get; set; }
public string Title { get; set; }
public string Author { get; set; }
public double Rating { get; set; }
}
Data Access Layer:
Create an IBookRepository interface with methods: Add(Book item), Remove(Book item), GetAll(), and GetById(int id).
Create a LibraryDbContext to set up the In-Memory Database. Also handle the Seeding of Books with a few examples.
Application Layer:
Create a BookService class that uses the IBookRepository to manage the books. Implement methods to AddBook, RemoveBook, ListAllBooks, and FindBookById.
Console UI Layer:
Create a menu-driven console application.
Options should include: Add a book, Remove a book, List all books, Find a book by ID, and Exit.
PRO Tip: the menu selection can be easily be implemented with a switch statement. However, we want to follow best coding practices right? 🙂
Think of a way to improve this part of the code. Using the Command Pattern could be a good way to go.
PRO Tip 2: Instead of using the Main method of Program.cs for doing all the work, you can also think of a way to implement Dependency Injection here, decoupling the different services that you will be using in the application. Not ideal for Console Apps but this is an exercise and we can enhance our practice session including more stuff 🙂
Docker
Now, once you have the Console App working properly, publish it in release mode into a folder, and use the app binaries to configure the dockerfile.
If you never used Docker before, you can read the official documentation Here. You can also browse on the internet or ask Chat-GPT, there are a ton of resources to learn the basics of Docker.
Once you have everything up and running, the main idea is to create a Docker image, and then run this image, deploying the application in a container. After running the container, you will be able to interactively use the menu to perform the different CRUD operations, all inside the container, completely isolated from your local environment. 😉
The Solution
There are multiple ways to achieve the resolution of this exercise, but as usual I’ll share with you a sample implementation which can be found under my Github profile:
Repo: https://github.com/marcelomusza/LibraryExerciseWithDocker
In order to maximize your learning opportunities throughout the course of this exercise, make sure to invest time in research whatever concept you are not familiar with, Google is your friend, and also Chat-GPT 😉
If you get completely stuck, then take a look to the working sample you’ll find under my Github profile. [...]
Read more...
1 August 2023Let’s create a WebAPI solution following some guidelines, in order to practice and improve our architecture skills, along with the understanding of the structure of the Application, and common features to be included.
If you are not familiar with Clean Architecture, I suggest you to read this article first:https://mulzatech.com/mastering-clean-architecture-in-net-a-comprehensive-guide-for-c-developers/
Clean Architecture C# Exercise
In this exercise you will create a blank and basic solution using the conventional project structure for the usage of Clean Architecture. You are tasked to add each project into the solution, add the required references for each one of them following the architecture standards.
When creating the Web API project you must enable support for Docker, and also use the template which contains the “WeatherController” boiler plate code, so we can easily compile the solution and make sure everything is working correctly.
Also recommended, but optional for this exercise, create a blank “Presentation” project which would be used to have the Frontend code of an application, if any. This layer could be used to hold a Web App using Blazor, for example, or perhaps could be removed completely in the case the web project is handled on a different solution using a different technology (such as Angular, next.js, etc)
You must also add the following nuget packages, and set them up correctly on the solution:
MediatR
FluentValidation
Serilog
And lastly, to practice your skills with git, you will create a blank repository on your Github personal profile (if you don’t have one, make sure to create it since it will become an extremely useful resource for your professional career!), and push the code there. Don’t forget to add the readme.md file!
And that’s it! The exercise will make you think about the correct solution structure, the different projects, the different layers and the connection between them. Also configuration of different nuget packages, including logging. And last but not least, you’ll use git to push the solution into your profile’s repository 😉
Proposed C# Exercise Challenges:
For this exercise I propose the following challenges to be considered, to maximize learning opportunities during our coding session.
Create a blank solution, so to add projects by hand as needed, and configure them properly, step by step.
Create a separate Dependency Injection class on each project, so we follow good coding practices at the time of the services registration. Then configure them properly on the WebAPI Project’s program.cs.TIP: MediatR and FluentValidation are services to be registered on the Application layer 😉
Configure Serilog on program.cs, and also provide the necessary settings on the configuration file for it to function properly. You can test this by running the WeatherController basic endpoint that is present when you create the WebAPI project.
Pay attention to good coding practices, and also the folder structure of your solution!
Get into the good habit of using git through the CLI, and publish the repo to your personal Github profile.
Solution
I’ll share below my suggested approach to structure this solution, so you can use as example if you get stuck at some point. Make sure to use Google whenever needed and also Chat-GPT 😉 before looking for the solution, so you enhance your problem solving skills.
Github Repo: https://github.com/marcelomusza/BaseCleanArchitectureExample/
Happy coding! [...]
Read more...